Pagination
A few of Maven's API utilize pagination patterns to return data back to the client. This is required because some requests may result in returning more data that can be returned in one call (or page). Below are a few pagination strategies Maven utilizes in its API suite.
Cursor (Time) Based Pagination
For APIs that deal with returning data that is time based, a cursor based pagination strategy is used. The expectation for endpoints that utilize cursor pagination is that a program is written that calls the endpoint in a loop.
With each call, the client program is passing in a time based cursor into the request to retrieve data for all records that have been generated after the cursor's value. This means that when calling the endpoint at time X, the cursor is set to X - Y where Y is the time your program sleeps between each call in the loop.
The cursor values need to be well formatted SO 8601 datetime strings. For example:
startTime=2024-01-01T17:01:00.000Z
A Practical Example
Suppose you are calling the GET /return-events
endpoint and your system calls the API every minute to retrieve return events.
The current date/time is 12:01 PM EST on January 1, 2024
To retrieve the return events for the past minute, you would make a call with the following startTime:
GET https://integrations.mavenmachines.com/return-events?startTime=2024-01-01T17:00:00.000Z
This will return all return events generated from the startTime of (12:00 PM) to now (12:01 PM)
After another minute passes and the time is 12:02, you would make a call to the endpoint with:
GET https://integrations.mavenmachines.com/return-events?startTime=2024-01-01T17:01:00.000Z
So on and so forth.
Dealing with multiple pages
In most cases, when calling an endpoint at a fixed interval that is small enough, you will receive all the data in one call. Sometimes if a lot of data is generated within a small time period or if your sleep time is large enough, there can exist more data to return that can fit in one page (one call).
Let's take the example below where we have 10 data elements we want to read from our sample API. In this case,t
= time and our API has a page size of 5 - which means a max of 5 data elements will be returned per call.

To call our API, we need to pass in our cursor which is query parameter called startTime
. For example:
https://integrations.mavenmachines.com/sample-api?startTime=1
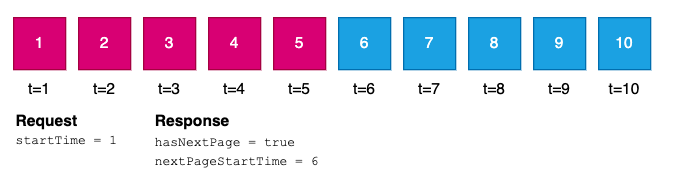
The response payload will include the 5 data elements along with a pagination block containing 2 fields. hasNextPage
is a boolean that tells the client if there is more data to receive and nextPageStartTime
is the value of the cursor for the next call.
{
"data": [1, 2, 3, 4, 5],
"pagination": {
"nextPageStartTime": 6,
"hasNextPage": true
}
}
To get the rest of our data, we need to make the following call:
https://integrations.mavenmachines.com/sample-api?startTime=6
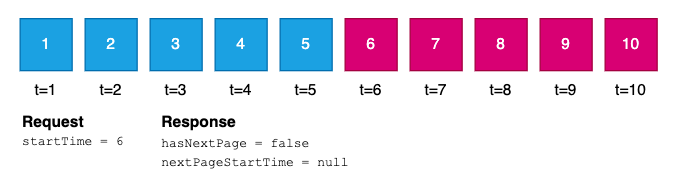
If hasNextPage
is false
this means that there is no more data to be retrieved and your program can return to normal operation.
Optional end time
APIs with cursor pagination also support an optional endTime parameter which means only return data up to this time. For example:
https://integrations.mavenmachines.com/sample-api?startTime=6&endTime=9
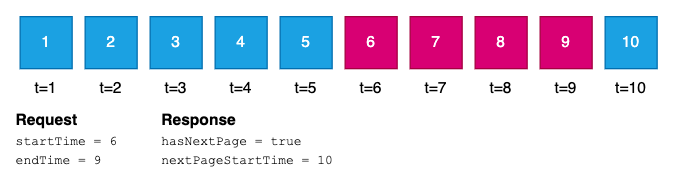
Implementation Notes
- All ISO 8601 strings are assumed to be in UTC.
- It is the client's responsibility to maintain the last known cursor position.
- Maven will only return a
nextPageStartTime
if there is more data to be returned / another page of data. - In almost all cases, the cursor time corresponds to when the data was uploaded into Maven's system vs when the actual event occurred (for example, in the case of a return event being generated or a vehicle ping being recorded). This is to ensure all data is returned in the case of a driver or a vehicle generating an event while being offline. This means that some records may appear out of order and needs to be handled accordingly.
Updated 2 months ago